Aws Lambda
Wed, Oct 18, 2017To create and deploy a python function to AWS Lambda involves uploading all the dependent code that goes with it.
The lambda calls the events endpoint in graphite which shows annotations in grafana. The trigger for me is going to be SNS.
Build your function locally
We need to package our code together with its dependent code. So we’re going to create a directory to keep it all in and download our dependencies to that directory. I’m using pip for this.
mkdir my-lambda
cd my-lambda
apt update
apt install wget python vim
wget https://bootstrap.pypa.io/get-pip.py
python get-pip.py
pip -V
pip install requests -t `pwd`
vim myfunction.py
The basic python code looks like this. We use the requests library to make a post to our graphite server to mark an event. Since the trigger is from AWS SNS, the json has an SNS wrapper which we’ll need to unwrap.
You can avoid this process by setting the SNS Topic as raw.
from __future__ import print_function
import json
import requests
print('Loading function')
def lambda_handler(event, context):
message = event['Records'][0]['Sns']['Message']
content = json.loads(message)
appName = content['applicationName']
state = content['state']
output = "%s has %s" % (appName, state)
print(output)
output = requests.post('http://graphite/events/', json={'what': output, 'tags' : [state]})
return event
Uploading to AWS Lambda
In terms of getting it in to aws, we need to zip it up and create the function. To maintain it we can just update the function.
This make file allows us to zip up the code package and create the initial lambda and then update it by just running make
deploy:
zip -r graphite-application-state.zip . -x Makefile -x graphite-application-state.zip
aws lambda update-function-code --function-name GraphiteApplicationStateChange --zip-file fileb://./graphite-application-state.zip
create:
zip -r graphite-application-state.zip . -x Makefile -x graphite-application-state.zip
aws lambda create-function --function-name="GraphiteApplicationStateChange" --runtime="python2.7" --role="arn:aws:iam::092013935146:role/service-role/GraphiteNotification" --handler="myfunc.lambda_handler" --zip-file fileb://./graphite-application-state.zip
So with this make file in the root directory, if the lambda doesn’t exist yet - just run
make create
after it has created, you can make changes as you see fit and just run
make
to update it.
Connecting to SNS
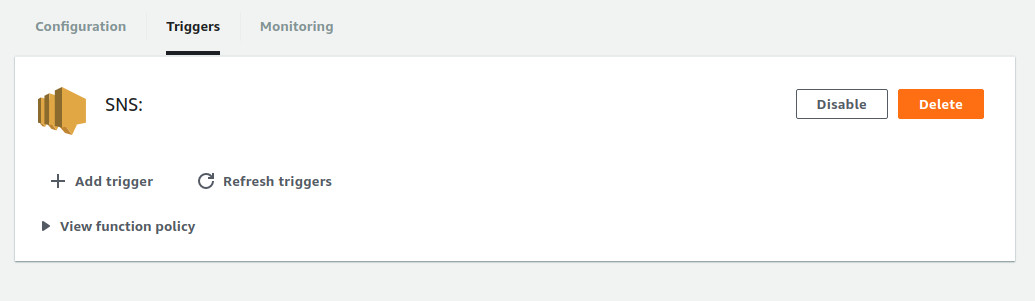
Add a trigger and you’ll get a selection for a variety of possible triggers. I’ve chosen SNS and then provided the arn for the topic i’m attaching.